Posting a Message
Gateway offers a JSON formatted RESTful API for the POSTing data into the platform. Each request and response follow a pre-set structure. The request is broken into; a header which provides a unique batch identifier and some additional control attributes; a payload which contain the main body of data being communicated. The payload element can receive multiple individual messages in a single POSTing.
The response is broken into three elements:
the data which provides a Gateway unique reference for the POST, as well as echoing back the third party’s batch reference and a time of receipt;
an errors element which provides information of issues encountered during the receipting process; and finally,
a success element which is a true or false value indicated if the call was POSTed successfully.
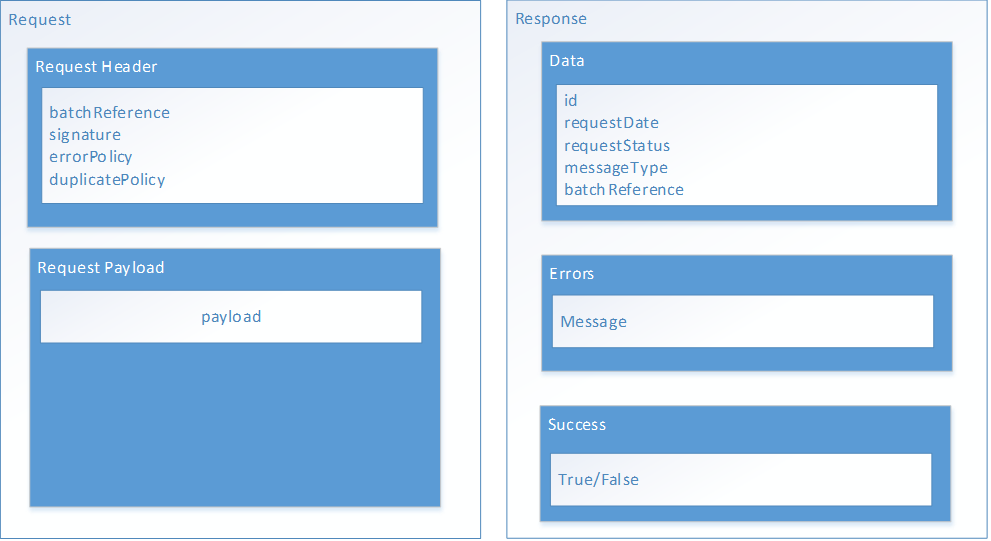
Request
A request is the submission of one of more messages to the Gateway platform. Each request is made up of the request header and payload sections. The request payload can contain one or more messages for processing. The attributes contained in a payload are determined by the message type and are validated against the schema(structure) of that type of message. The header contains the following attributes:
batchReference - Mandatory. This is the clients’ reference number. This allows clients to map requested messages back to their reference number.
errorPolicy - This indicates how the processing of the remaining batch items will be conducted in the event of an item failing.
haltOnError (default): marks the failed item as ‘failed’ and terminates processing, leaving any unprocessed items in a state of ‘cancelled’.
proceedOnError: marks the failed item as ‘failed’ and continues to process any remaining items in the batch.
duplicatePolicy - This indicates how the processing of the requests and messages are conducted in the event of duplicate. Gateway uses the external reference in a message to identify is a similar entity record has previously been received.
skipDuplicates (default): will not attempt to process any message that has an external reference that has previously been processed. The message will be skipped and set to a ‘cancelled’ state.
processDuplicates: will attempt to re-process messages with the same external reference and will attempt to update the previously received message (depending on its current state)
signature: Optional attribute reserved for future use. It is intended to be a digital signature of the entire payload field, to help ensure data integrity.
Message Updating
Some messages have a hierarchy provide sub-lists or collections of other items that are not classified as a message type in their own right (phone numbers for example). When the duplicatePolicy is set to processDuplicates, all such sub-lists (during the update process) will be deleted, and the new list supplied will replace them.
Once a request has been received, authenticated, authorised and validated for basic format syntax. It is accepted and placed in a queue for processing. When the request is selecting for processing into the gateway platform the individual message are unpacked are further validate, where routing, transformation and other pre-processing activities take place. The message(s) are then sent to the destination service for processing. Once successfully, receipted the request and its individual message payloads will be update providing a status and details of an issues during the injection process. A detailed description of the injection process is a follows:
New request POST’ed
HTTP request is authenticated
If Authentication fails, the request is rejected with HTTP Return Code = 401 - Unauthorized
Authority to use the requested API endpoint
If the client is not Authorized to this message type, the request is rejected with HTTP Return Code = 403 – Forbidden
Request is validated
The fields in the root of the request are validated
Individual message types contained within the payload are not validated at this time
If validation fails, the request is rejected with HTTP Return Code = 400 – Bad Request.
Request is Accepted
Request is queued for processing
The requestStatus = Queued
The request can now be tracked through the Tracking API endpoint
HTTP Return Code = 200 – Ok
Each accepted request is processed as a batch.
Individual messages contained within the request are split out for processing
The requestStatus = Processing
Each individual messageStatus will show as messageStatus = Queued
The message payload is validated against the individual message type schema
Validation typically includes:
Mandatory fields
Field type checking
Enumeration checking
If Validation passes:
Message sent for processing
Message status is set to messageStatus = Processing
Duplicate checks are performed to determine if the message should be processed
If the externalReference has not been previously sent in a message, the message is processed as an INSERT operation
If the externalReference has been previously sent and duplicatePolicy = processDuplicates, the message is processed as an UPDATE operation
If the message was processed successfull:, messageStatus = Complete
If the message was not processed: messageStatus = Cancelled
If there was an error while processing: messageStatus = Failed
Remaining messages in the Request are processed in the same way, until all are processed, and the Request status is set to: requestStatus = Complete (if success for all messages), requestStatus = Failedd (if one or more messages are not success)
If validation or processing of a message fails:
The message is updated with an error code and description, as well as the message status being set to messageStatus = Failed (if a processing issue) or messageStatus = Invalid (if validation issue)
If there is a single message in the Request, processing is complete, and the Request status is set to requestStatus = Failed
If other messages are contained in the Request and the errorPolicy = proceedOnError, the next message will be processed.
If other messages are contained in the Request and the errorPolicy = haltOnError, all unprocessed messages in the Request are set to messageStatus = Cancelled and processing ends immediately by setting the Request status to requestStatus = Partial
At the end of Request processing, if any messages have failed and the errorPolicy = proceedOnError, then the Request status is set to requestStatus = Partial, and processing ends.
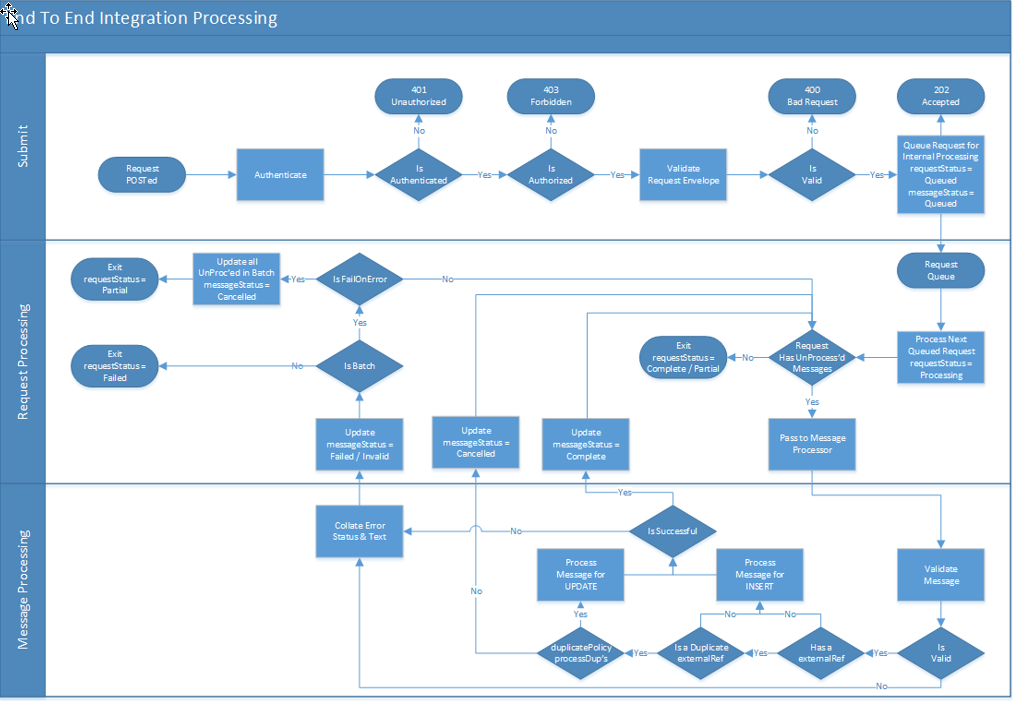
Request Response
Gateway APIs provides a POST response to every Request made to the platfrom. This response will include a stand HTTP status code as well as a data payload which provides addtional information about the status or error details. The attribute of a Gateway response message are as follows:
Data - Data object containing response details
Id - A unique ID (GUID) allocated to a Request by the Gateway platfrom. This ID can be used to query the status of a Request after it has been receipted, using the Tracking API.
requestDate - The date and time the Request was received by the Gateway API service.
requestStatus - The processing status of a Request. This status is Queued when the Request is first received. Other possible states are:
Processing – Gateway is in the process of storing the payload messages
Partial – One or more of the payload messages have not been stored successfully
Complete – All payload messages have been stored successfully
Failed – The Request and payload messages have not been stored
batchReference - An echo back of the caller’s unique identifier passed in the header of the Request
errors - An array of errors, descriptions and codes, that may have occurred during processing.
success - A boolean true or false value indicating if the Request was received correctly.
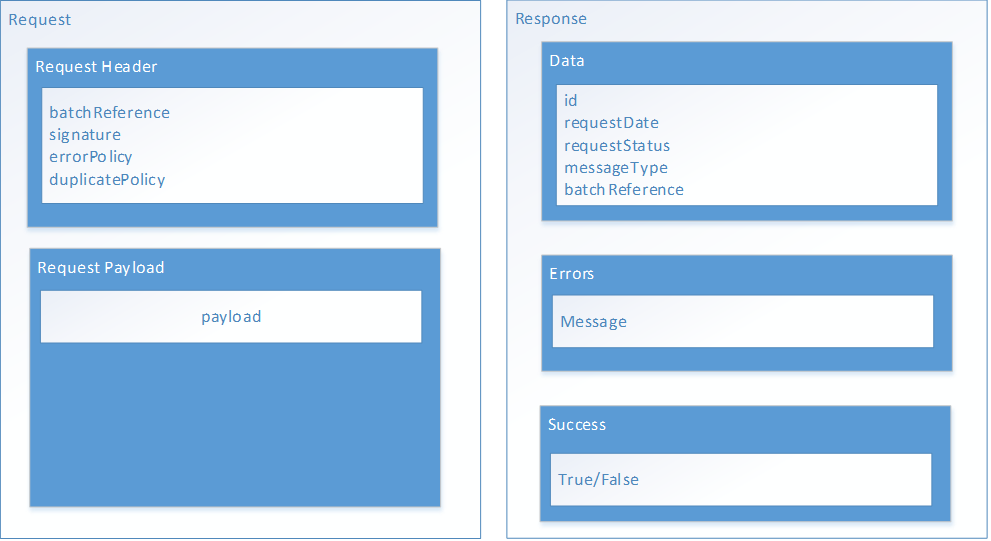
Late binding
Gateway supports the late-binding of entities (messages). This prevents the need for sequencing or dependencies on the order in which messages are received and processed. This design promotes the use of smaller (fine grain) messages over large monolithic hierarchal message structures and fits nicely into a micro service architecture.
For example, if a message references several different entities (other parties, vehicles, ...), and if this referencing message is processed before the other vehicle or party messages, it will not fail. When the party and vehicle messages are processed, the referencing entity will automatically be updated to show the data held in these newly created entities.
The late-binding process is managed through the externalReference attribute (which every message contains). For late binding to work each message must include an externalReference value and it must be a globally unique identifier for that data entity.
In the event of a message referencing an entity (externalReference) that has not yet been created in the platform, a placeholder will be created for the unknown entity.
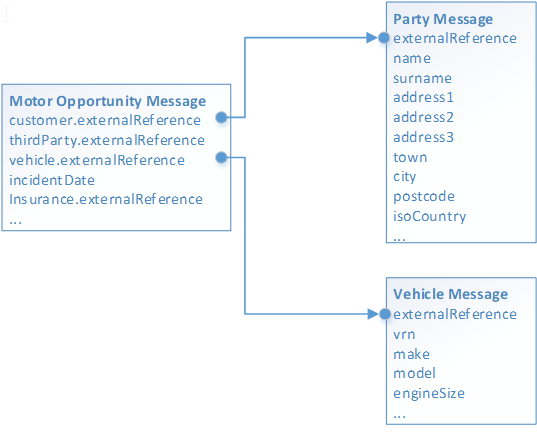
Standard data format conventions
Dates and time
Date and times (where appropriate) will be represented in ISO 8601 format, as follows:
yyyy-mm-ddThh:mm:ss[.mmm]±hh:mm
Examples:
2019-01-25T14:55:30Z
2019-01-25T14:55:30Z
2019-01-25T14:55:30Z
Decimals
Decimals will be represented as follows:
0.0000
With a numeric scale of up to 4 decimal places, unless otherwise stated.
Booleans
Booleans should be in the form of either true or false.
Examples:
"isVATRegistered": true Correct
"isVATRegistered": false Correct
"isVATRegistered": "false" Incorrect
"isVATRegistered": "No" Incorrect
"isVATRegistered": "0" Incorrect
Enumerations
Enumerations are listed in the API definition. The value will always be a number (except in case of country codes).
Examples:
"insuranceCoverType": "Comprehensive" Incorrect
"insuranceCoverType": 0 Correct